Admin 项目实战(五):按需引入
这是 Admin 练习项目的第五篇,练习如何通过插件实现自动引入项目用到的 API 和组件。
安装与配置插件
安装 unplugin-auto-import 与 unplugin-vue-components 插件:
| pnpm i unplugin-auto-import -D
pnpm i unplugin-vue-components -D
|
在 Vite 中进行配置:
| // vite.config.ts
import AutoImport from "unplugin-auto-import/vite";
import Components from "unplugin-vue-components/vite";
export default defineConfig({
//...
plugins: [
//...
AutoImport({
imports: ["vue", "vue-router", "pinia"],
dts: "src/auto-imports.d.ts",
}),
Components({
extensions: ["vue"],
include: [/\.vue$/],
dts: "src/components.d.ts",
}),
],
});
|
上面的配置中,
- 自动导入 vue、vue-router、pinia 的 API 到
src/auto-imports.d.ts
;
- 自动加载 vue 文件中的组件到
src/components.d.ts
。
按需引入 API
配置前的代码
| import { ref } from "vue";
const num = ref(0);
const add = () => {
num.value++;
};
|
配置后的代码
| const num = ref(0);
const add = () => {
num.value++;
};
|
实现原理
在生成的 src/auto-imports.d.ts
文件中,会自动检测并进行全局引入:
| // src/auto-imports.d.ts
export {}
declare global {
//..
const ref: typeof import('vue')['ref']
}
// for type re-export
declare global {
// @ts-ignore
export type { Ref, /*...*/ } from 'vue'
}
|
运行效果
启动项目可以正常访问 index ,点击按钮可以正确增加计数值。
按需引入组件
准备工作
新建 components 目录并加入 Card 组件,card.vue:
| <template>
<div class="shadow-lg rounded-2xl w-36 p-4 bg-white dark:bg-gray-800">
<div class="flex items-center">
<span class="bg-green-500 p-2 h-4 w-4 rounded-full relative">
<svg
width="20"
fill="currentColor"
height="20"
class="text-white h-2 absolute top-1/2 left-1/2 transform -tran slate-x-1/2 -translate-y-1/2"
viewBox="0 0 1792 1792"
xmlns="http://www.w3.org/2000/svg"
>
<path
d="M1362 1185q0 153-99.5 263.5t-258.5 136.5v175q0 14-9 23t-23 9h-135q-13 0-22.5-9.5t-9.5-22.5v-175q-66-9-127.5-31t-101.5-44.5-74-48-46. 5-37.5-17.5-18q-17-21-2-41l103-135q7-10 23-12 15-2 24 9l2 2q113 99 243 12 5 37 8 74 8 81 0 142.5-43t61.5-122q0-28-15-53t-33.5-42-58.5-37.5-66-32-80 -32.5q-39-16-61.5-25t-61.5-26.5-62.5-31-56.5-35.5-53.5-42.5-43.5-49-35.5- 58-21-66.5-8.5-78q0-138 98-242t255-134v-180q0-13 9.5-22.5t22.5-9.5h135q14 0 23 9t9 23v176q57 6 110.5 23t87 33.5 63.5 37.5 39 29 15 14q17 18 5 38l-8 1 146q-8 15-23 16-14 3-27-7-3-3-14.5-12t-39-26.5-58.5-32-74.5-26-85.5-11. 5q-95 0-155 43t-60 111q0 26 8.5 48t29.5 41.5 39.5 33 56 31 60.5 27 70 27. 5q53 20 81 31.5t76 35 75.5 42.5 62 50 53 63.5 31.5 76.5 13 94z"
></path>
</svg>
</span>
<p class="text-md text-gray-700 dark:text-gray-50 ml-2">Sales</p>
</div>
<div class="flex flex-col justify-start">
<p
class="text-gray-800 text-4xl text-left dark:text-white font-bol d my-4"
>
36K
</p>
<div class="relative w-28 h-2 bg-gray-200 rounded">
<div
class="absolute top-0 h-2 left-0 rounded bg-green-500 w-2/ 3"
></div>
</div>
</div>
</div>
</template>
|
在 index.vue 中加入 card 组件:
| <template>
<h1>Index Page</h1>
<card></card>
</template>
|
运行效果
启动项目可以正常访问 index ,可以看到 Card 组件自动加载成功。
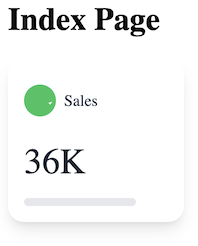
![]()
实现原理
在生成的 src/components.d.ts
文件中,会自动检测并加载组件:
| import '@vue/runtime-core'
export {}
declare module '@vue/runtime-core' {
export interface GlobalComponents {
Card: typeof import('./components/Card.vue')['default']
RouterLink: typeof import('vue-router')['RouterLink']
RouterView: typeof import('vue-router')['RouterView']
}
}
|
上一课
Admin 项目实战(六):可配置布局管理
凡本网注明"来源:XXX "的文/图/视频等稿件,本网转载出于传递更多信息之目的,并不意味着赞同其观点或证实其内容的真实性。如涉及作品内容、版权和其它问题,请与本网联系,我们将在第一时间删除内容!
作者: 勃纳什
来源: https://zhuanlan.zhihu.com/p/644430977